Top 10 Arduino Projects for Beginners
Student Project Ideas
EDUCATIONAL PROJECTS
7/14/20245 دقيقة قراءة
Top 10 Arduino Projects for Beginners
Introduction
Arduino is a powerful open-source platform that simplifies the process of creating electronic projects. With its easy-to-use hardware and software, it's an ideal choice for beginners looking to dive into the world of electronics and programming. Here are ten beginner-friendly Arduino projects that will help you get started and build a solid foundation in electronics.
1. Blinking LED
Overview: The classic "Hello World" of Arduino projects, this project will teach you how to control an LED using an Arduino board.
Components:
- Arduino board (e.g., Arduino Uno)
- LED
- 220-ohm resistor
- Breadboard
- Jumper wires
Steps:
1. Connect the LED to a digital pin (e.g., pin 13) on the Arduino board.
2. Place the 220-ohm resistor in series with the LED.
3. Write and upload the following code to the Arduino:
```cpp
void setup() {
pinMode(13, OUTPUT); // Set pin 13 as an output
}
void loop() {
digitalWrite(13, HIGH); // Turn the LED on
delay(1000); // Wait for a second
digitalWrite(13, LOW); // Turn the LED off
delay(1000); // Wait for a second
}
```
2. Temperature Sensor
Overview: Learn how to read temperature data from a sensor and display it using the Arduino.
Components:
- Arduino board
- LM35 temperature sensor
- Breadboard
- Jumper wires
Steps:
1. Connect the LM35 sensor to the Arduino (VCC to 5V, GND to GND, and OUT to an analog input pin, e.g., A0).
2. Write and upload the following code:
```cpp
int tempPin = A0;
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
int tempReading = analogRead(tempPin); // Read the analog input
float voltage = tempReading * 5.0 / 1024; // Convert to voltage
float temperatureC = voltage * 100; // Convert to Celsius
Serial.print("Temperature: ");
Serial.print(temperatureC);
Serial.println(" C");
delay(1000); // Wait for a second
}
```
3. Light Sensor
Overview: Measure the ambient light levels using a photoresistor and display the values.
Components:
- Arduino board
- Photoresistor (LDR)
- 10k-ohm resistor
- Breadboard
- Jumper wires
Steps:
1. Connect the photoresistor to the Arduino (one side to 5V and the other to an analog input pin, e.g., A0, with a 10k-ohm resistor to GND).
2. Write and upload the following code:
```cpp
int lightPin = A0;
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
int lightReading = analogRead(lightPin); // Read the analog input
Serial.print("Light level: ");
Serial.println(lightReading);
delay(1000); // Wait for a second
}
```
4. RGB LED Control
Overview: Create different colors by controlling an RGB LED.
Components:
- Arduino board
- RGB LED
- 220-ohm resistors
- Breadboard
- Jumper wires
Steps:
1. Connect the RGB LED to the Arduino (common cathode to GND, and the R, G, B pins to digital pins with resistors).
2. Write and upload the following code:
```cpp
int redPin = 9;
int greenPin = 10;
int bluePin = 11;
void setup() {
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
setColor(255, 0, 0); // Red
delay(1000);
setColor(0, 255, 0); // Green
delay(1000);
setColor(0, 0, 255); // Blue
delay(1000);
}
void setColor(int red, int green, int blue) {
analogWrite(redPin, red);
analogWrite(greenPin, green);
analogWrite(bluePin, blue);
}
```
5. Push Button Control
Overview: Learn how to read input from a push button and control an LED.
Components:
- Arduino board
- Push button
- 10k-ohm resistor
- LED
- 220-ohm resistor
- Breadboard
- Jumper wires
Steps:
1. Connect the push button to the Arduino (one side to 5V, the other side to a digital pin with a pull-down resistor to GND).
2. Connect the LED to another digital pin.
3. Write and upload the following code:
```cpp
int buttonPin = 2;
int ledPin = 13;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
int buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
digitalWrite(ledPin, HIGH); // Turn the LED on
} else {
digitalWrite(ledPin, LOW); // Turn the LED off
}
}
```
6. Buzzer Alarm
Overview: Create a simple alarm system using a buzzer and a motion sensor.
Components:
- Arduino board
- Passive buzzer
- PIR motion sensor
- Breadboard
- Jumper wires
Steps:
1. Connect the PIR sensor to the Arduino (VCC to 5V, GND to GND, and OUT to a digital pin, e.g., pin 2).
2. Connect the buzzer to another digital pin (e.g., pin 8).
3. Write and upload the following code:
```cpp
int pirPin = 2;
int buzzerPin = 8;
void setup() {
pinMode(pirPin, INPUT);
pinMode(buzzerPin, OUTPUT);
}
void loop() {
int pirState = digitalRead(pirPin);
if (pirState == HIGH) {
digitalWrite(buzzerPin, HIGH); // Turn the buzzer on
} else {
digitalWrite(buzzerPin, LOW); // Turn the buzzer off
}
}
```
7. Servo Motor Control
Overview: Control the position of a servo motor using a potentiometer.
Components:
- Arduino board
- Servo motor
- Potentiometer
- Breadboard
- Jumper wires
Steps:
1. Connect the potentiometer to the Arduino (VCC to 5V, GND to GND, and the wiper to an analog input pin, e.g., A0).
2. Connect the servo motor to a digital pin (e.g., pin 9).
3. Write and upload the following code:
```cpp
#include <Servo.h>
Servo myservo;
int potPin = A0;
void setup() {
myservo.attach(9); // Attach servo to pin 9
}
void loop() {
int potValue = analogRead(potPin);
int angle = map(potValue, 0, 1023, 0, 180); // Map potentiometer value to servo angle
myservo.write(angle); // Set servo angle
delay(15);
}
```
8. Ultrasonic Distance Sensor
Overview: Measure distances using an ultrasonic sensor and display the values.
Components:
- Arduino board
- HC-SR04 ultrasonic sensor
- Breadboard
- Jumper wires
Steps:
1. Connect the HC-SR04 sensor to the Arduino (VCC to 5V, GND to GND, Trig to a digital pin, and Echo to another digital pin).
2. Write and upload the following code:
```cpp
const int trigPin = 9;
const int echoPin = 10;
void setup() {
Serial.begin(9600);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
long duration = pulseIn(echoPin, HIGH);
int distance = duration * 0.034 / 2;
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(1000);
}
```
9. LCD Display
Overview: Display messages on an LCD screen.
Components:
- Arduino board
- 16x2 LCD display
- 10k-ohm potentiometer
- Breadboard
- Jumper wires
Steps:
1. Connect the LCD to the Arduino following the standard wiring for a 16x2 LCD.
2. Write and upload the following code:
```cpp
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
lcd.begin(16, 2); // Set up the LCD's number of columns and rows
lcd.print("Hello, World!"); // Print a message
}
void loop() {
// Nothing to do here
}
```
10. Traffic Light System
Overview: Simulate a traffic light system using LEDs.
**Components
:**
- Arduino board
- Red, yellow, and green LEDs
- 220-ohm resistors
- Breadboard
- Jumper wires
Steps:
1. Connect the LEDs to the Arduino (each LED to a digital pin with resistors).
2. Write and upload the following code:
```cpp
int redPin = 13;
int yellowPin = 12;
int greenPin = 11;
void setup() {
pinMode(redPin, OUTPUT);
pinMode(yellowPin, OUTPUT);
pinMode(greenPin, OUTPUT);
}
void loop() {
digitalWrite(redPin, HIGH);
delay(5000);
digitalWrite(redPin, LOW);
digitalWrite(yellowPin, HIGH);
delay(2000);
digitalWrite(yellowPin, LOW);
digitalWrite(greenPin, HIGH);
delay(5000);
digitalWrite(greenPin, LOW);
}
```
Conclusion
These ten projects provide a comprehensive introduction to Arduino and its capabilities. By working through these projects, you'll gain valuable experience in programming, electronics, and project design, setting a solid foundation for more advanced projects in the future. Happy building!
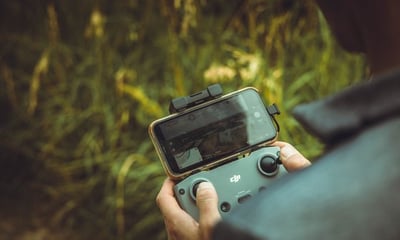
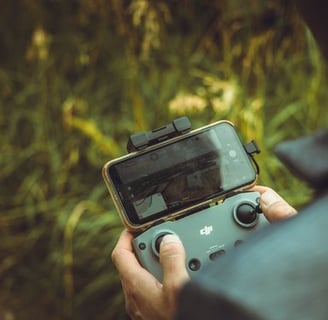

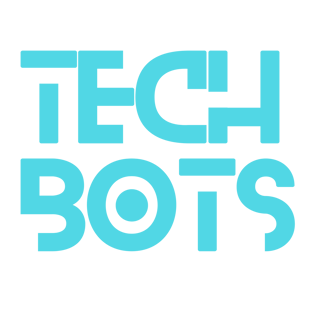
Robotics News
TechBots
20 JULY 2024 4 MIN READ
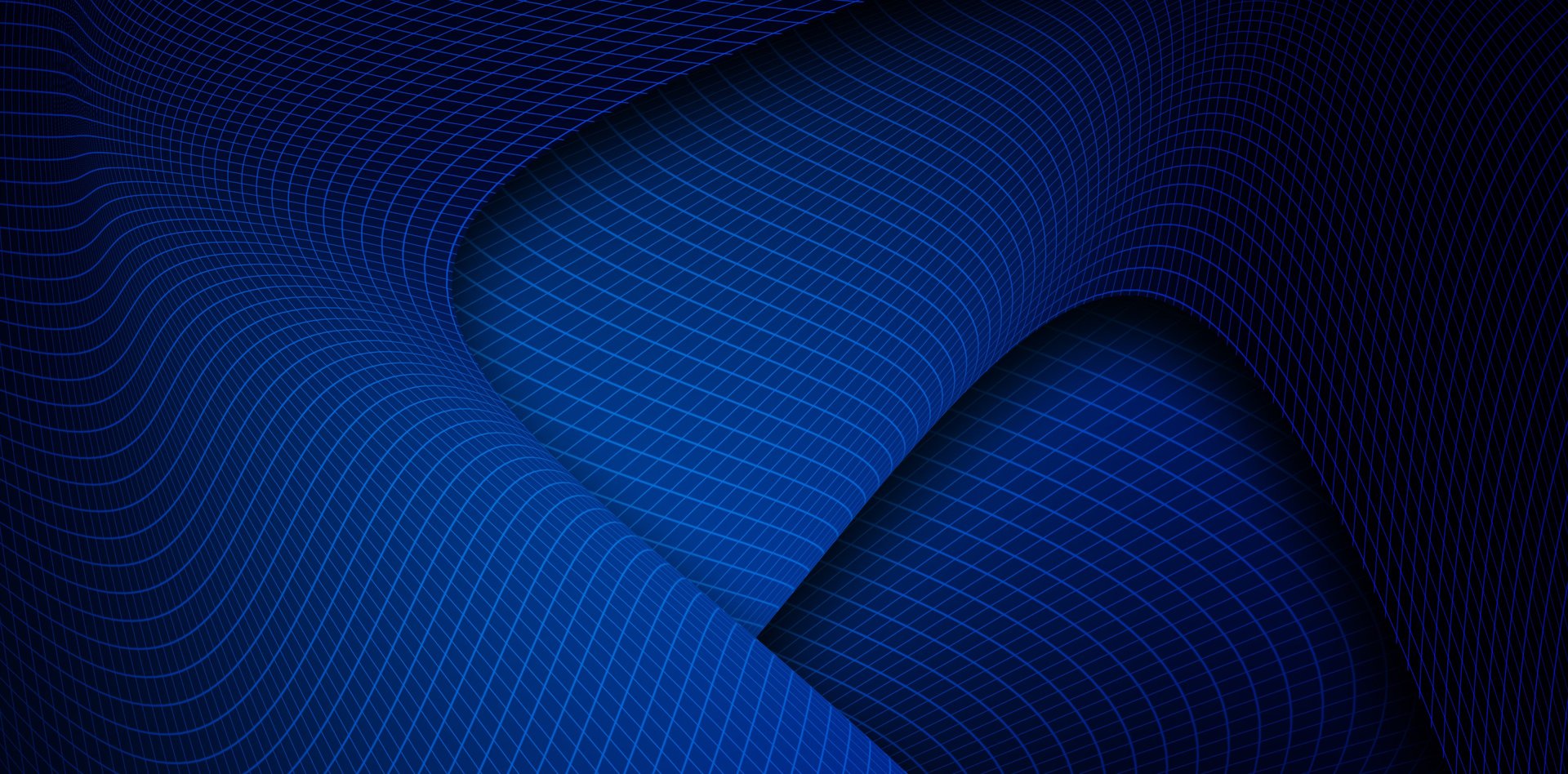